|
In the realm of modern software development, creating APIs (Application Programming Interfaces) is essential for facilitating communication between different software systems. In this blog post, we’ll delve into the process of creating APIs in C# using the ASP.NET framework.
We’ll cover the basics of API development, including the implementation of HTTP methods such as GET, POST, PUT, and DELETE. Additionally, we’ll explore the distinctions between APIs and web services, and provide a practical example that can be tested using Postman.
Let’s dive in!
APIs (Application Programming Interfaces) allow different software systems to communicate with each other. APIs work by exposing endpoints that can be called by other programs. In this post, we’ll look at how to create a basic API using C# and ASP.NET Core.
What is an API?
An API is a set of definitions and protocols for building and integrating application software. APIs let your product or service communicate with other products and services without having to know how they’re implemented. This allows you to open up data and functionality to other developers and to other systems.
APIs allow you to securely expose certain parts of your application logic to the outside world in a controlled manner. For example, the Twitter API allows other developers to integrate Twitter data into their own applications, under certain conditions. APIs are the backbone of the interconnected web services we use every day.
Understanding APIs and Web Services
Before we delve into the technical aspects of API development, it’s crucial to grasp the distinction between APIs and web services. While both enable communication between software systems, they differ in their implementation and scope.
APIs serve as interfaces that allow different software applications to interact with each other. They define the methods and protocols that developers can use to access the functionalities of a software system.
Web services, on the other hand, are a specific type of API that operates over the web using standard protocols such as HTTP. They typically follow the principles of REST (Representational State Transfer) or SOAP (Simple Object Access Protocol).
Setting up the Development Environment
To create APIs in C#, you’ll need the following prerequisites:
- Visual Studio Code IDE: To code any software you need an IDE. One of the most popular integrated development environment (IDE) for building .NET applications is Visual Studio Code. You can install the application by going to the official VS Code link from Microsoft.
- ASP.NET Core SDK: Ensure that you have the ASP.NET Core SDK installed, as it provides the necessary tools for developing web applications with C#. You can download it from the official .NET link from Microsoft.
- Postman: Finally, download and install Postman, a popular API development and testing tool. You’d need Postman to call the various endpoints that we will code in this tutorial. You can download Postman from the official link.
- Basic knowledge of C# and .NET Framework is needed.
It is important to note that VS Code doesn’t include a traditional File > New Project dialog or pre-installed project templates. You’ll need to add additional components and scaffolders depending on your development interests.
Pro-Tip: You can install the C# Dev Kit within VS Studio Code to test your code with integrated unit test discovery and execution
Creating the API Project
Let’s start by creating a new ASP.NET Web Application project in Visual Studio Code:
- Create a New Folder: Create a new folder for your C# project using Visual Studio Code’s file explorer or in your terminal/command prompt.
- Initialize a new C# Project: Open the terminal in Visual Studio Code (press `Ctrl+“) and navigate to your project folder. You can also navigate to Terminal option on the top ribbon of your VS code and click on New Terminal. Run the following command to initialize a new C# project:
dotnet new webapi -n MyApiProject
This will create a new project with the required references and packages needed to build a basic Web API. The folder structure should look something like below:
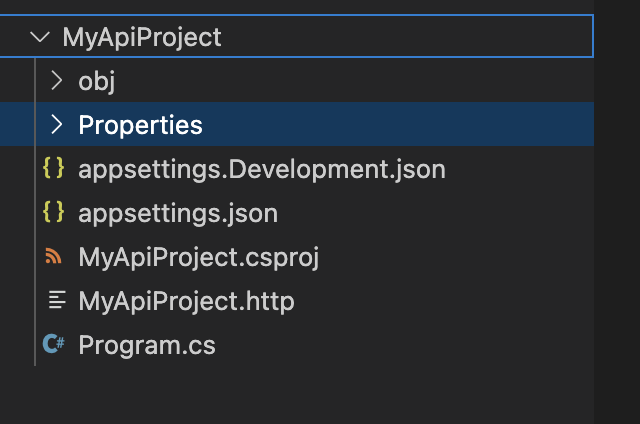
Adding a Model
Under the project folder, create a new Models
folder and add a Product.cs
class:
Now let’s add a simple Product
model that we will use in our API:
namespace MyApiProject.Models
{
public class Product
{
public Product()
{
Name = "";
}
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
}
This Product
class will act as our data model that we will perform CRUD operations on.
Creating the Controller
The main logic of our API will reside in the controller. Let’s add a ProductsController
under the Controllers folder:
using Microsoft.AspNetCore.Mvc;
using MyApiProject.Models;
namespace MyApiProject.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ProductsController : ControllerBase
{
// Controller methods (We will add the CRUD Logic here)
}
}
We decorate the class with [Route]
and [ApiController]
attributes to mark it as a controller that will handle API requests.
Adding CRUD Methods
Now let’s add methods for CRUD operations:
Get All Products
GET Method – The GET method in API is used to request data from a specified resource. When a client sends a GET request to a server, it is asking for information from the server. This method is used for retrieving data and should not have any other effect on the data or server state.
GET requests are typically used for operations such as reading or fetching data, and they do not have a request body. In RESTful APIs, GET requests are often used to retrieve resource representations, such as retrieving a list of items or fetching a specific item by its unique identifier.
Let’s continue with the example of adding a simple GET method to get Products
// GET: api/products
[HttpGet]
public IActionResult GetProducts()
{
// Return list of products (dummy data)
return Ok(new List<Product>
{
new Product { Id = 1, Name = "Apple", Price = 1.99M },
new Product { Id = 2, Name = "Orange", Price = 2.99M },
});
}
This method handles GET request to /api/products
and returns a list of products.
Get Single Product
// GET: api/products/5
[HttpGet("{id}")]
public IActionResult GetProduct(int id)
{
// Return single product (dummy data)
var product = new Product { Id = id, Name = "Tomato", Price = 3.99M };
return Ok(product);
}
This method takes an id
parameter from route and returns a single product object.
Create Product
Post Method – The POST method is used to submit data to be processed to a specified resource. When a client sends a POST request to a server, it is requesting the server to accept and store the enclosed entity, usually as a new resource or as an update to an existing resource.
POST requests often include a request body containing data that the server needs to process. Common use cases for POST requests include creating new resources, submitting form data, and performing actions that result in state changes on the server.
// POST: api/products
[HttpPost]
public IActionResult CreateProduct([FromBody] Product product)
{
// Create product logic here
return CreatedAtAction(nameof(GetProduct), new { id = product.Id }, product);
}
Here we handle POST request, take Product
object from request body, create product and return 201 response.
Update Product
Put Method: The PUT method is used to update or replace a resource identified by a specific URI (Uniform Resource Identifier). When a client sends a PUT request to a server, it is requesting the server to update the resource at the given URI with the enclosed entity in the request body.
PUT requests are idempotent, meaning that multiple identical requests should have the same effect as a single request. PUT requests are commonly used for updating existing resources with new data provided in the request body.
// PUT: api/products/5
[HttpPut("{id}")]
public IActionResult UpdateProduct(int id, [FromBody] Product product)
{
// Update product logic here
return NoContent();
}
This method handles PUT request, takes id
from route and updated Product
object from body and updates the product.
Delete Product
DELETE Method: The DELETE method is used to request the removal of a specific resource from the server. When a client sends a DELETE request to a server, it is asking the server to delete the resource identified by the specified URI.
DELETE requests are idempotent, meaning that making the same request multiple times should have the same effect as a single request. DELETE requests are typically used to delete resources that are no longer needed or to perform cleanup operations.
// DELETE: api/products/5
[HttpDelete("{id}")]
public IActionResult DeleteProduct(int id)
{
// Delete product logic here
return NoContent();
}
This method handles DELETE request, takes id
from route and deletes the product.
That completes the basic CRUD methods for our API!
Here is the complete code for ProductsController.cs with some sample data
using Microsoft.AspNetCore.Mvc;
using MyApiProject.Models;
namespace MyApiProject.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ProductsController : ControllerBase
{
// GET All
[HttpGet]
public IActionResult GetProducts()
{
return Ok(GetSampleProducts());
}
// GET by Id
[HttpGet("{id}")]
public IActionResult GetProduct(int id)
{
return Ok(GetSampleProducts().FirstOrDefault(x => x.Id == id));
}
// POST
[HttpPost]
public IActionResult CreateProduct([FromBody] Product product)
{
// Save product
return CreatedAtAction(nameof(GetProduct), new { id = product.Id }, product);
}
// PUT
[HttpPut("{id}")]
public IActionResult UpdateProduct(int id, [FromBody] Product product)
{
// Update product
return NoContent();
}
// DELETE
[HttpDelete("{id}")]
public IActionResult DeleteProduct(int id)
{
// Delete product
return NoContent();
}
// Sample data
List<Product> GetSampleProducts()
{
return new List<Product>()
{
new Product() { Id = 1, Name = "Apple", Price = 1.50M },
new Product() { Id = 2, Name = "Orange", Price = 2.50M },
};
}
}
}
Changes to Program.cs
Finally, add code to set up the web host and map the Controllers for routing.
using Microsoft.AspNetCore.Mvc;
using MyApiProject.Models;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
var app = builder.Build();
// Configure the HTTP request pipeline.
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
Build and Run
Before we test the API, let’s do a build and run. Build compiles the code and checks for the errors, run command starts the localhost server.
- Use
dotnet build
to compile code - Use
dotnet run
to start local server
When do do the run, remember to check the terminal to see which port the localhost is using. This can be changed in your project in the launchSettings.json file. For example, my project is using port 5164 by default.
Testing the API
Let’s test the API quickly using Postman.
- Launch the API project from Visual Studio. This will run the API at
http://localhost:{port}/api/products
- Open Postman and create a new request.
- Select GET method and enter the URL
http://localhost:{port}/api/products
to test GetProducts API. This should return a list of products. - Similarly, you can test other APIs by passing appropriate URLs and parameters.
And that’s it! In this way, you can quickly build and test a basic Web API in C# and .NET Framework using Visual Studio.
Conclusion
In this blog post, we’ve explored the process of creating APIs in C# using ASP.NET Core. We’ve covered the basics of API development, explained the differences between APIs and web services, and provided a practical example with CRUD operations for a book API.
By following these steps and leveraging the power of C# and ASP.NET Core, you can develop robust APIs to facilitate seamless communication between your software systems.
Happy coding!
Further Reading:
AI for Absolute Beginners: No Coding Required
Navigating the Job Market: Most In-Demand Tech Skills in 2024